Mastering Material Usage Optimization For Your Projects - A Python-Powered Solution
This article was originally published on July 15, 2023 on linkedin.com
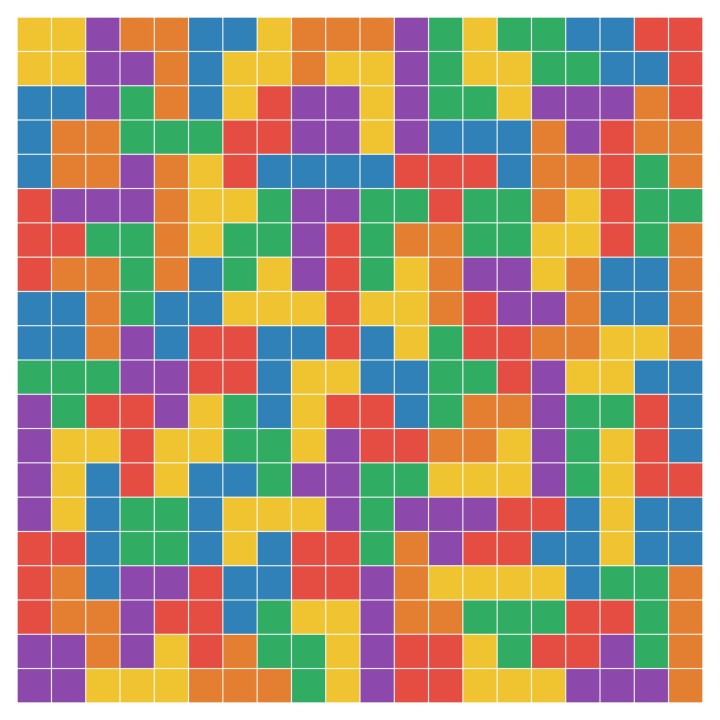
Tetris Vectors by Vecteezy: https://www.vecteezy.com/free-vector/tetris
Intro
In the previousĀ post, I shared links to some ready-to-use online calculators that provide a quick tool for finding the optimal amount of materials to order for your project. However, what if you want to add additional features to these calculators or work around limitations like the number of parts? In this article, I will show you how to write Python code to solve this problem and create your own flexible solution.
To illustrate the process, we will use the example of a bar schedule for concrete foundations, where we need to cut rebar with specific lengths and quantities.
We use some sample data
Part 1. Quick Start. Optimizing the required number of rebarĀ
Letās start by calculating the number of rods to order based on the demand indicated in the table. We can convert the table into a list of required rebar parts, repeating each element as many times as indicated in the table. In this case, we have a list of 25 elements.
parts = [2350, 2350, 2350, 2350, 2800, 3030, 3030, 3030, 3030, 3030, 3030, 3030, 3030, 3030, 3030, 3030, 3030, 3030, 3030, 4230, 4230, 4230, 4230, 4230, 4230]
Next, we can use theĀ ābinpackingāĀ Ā package to solve the problem in just one line of code:
rods = binpacking.to_constant_volume(parts, STOCK_REBAR_LENGTH)
By adding print functions to display the results, we can see the required number of bars and the cutting scheme:
import binpacking
STOCK_REBAR_LENGTH = 11700
rods = binpacking.to_constant_volume(parts, STOCK_REBAR_LENGTH)
print(f'Required number of bars: {len(rods)} pcs')
print(f'Cutting scheme: {rods}')
The output will show the required number of bars and the cutting scheme for the rebar:
Required number of bars: 7 pcs
Cutting scheme: [[4230, 4230, 3030], [4230, 4230, 3030], [4230, 4230, 3030], [3030, 3030, 3030, 2350], [3030, 3030, 3030, 2350], [3030, 3030, 3030, 2350], [3030, 3030, 2800, 2350]]
Although the blade thickness is not taken into account (which can be neglected in our case) and the variance of rebar in stock cannot be defined (as we assume only one length of 11700 mm), we have managed to find the optimal amount of rebar to order and determine the cutting scheme with just a few lines of code..
Part 2. Optimization of the required quantity of reinforcement for the entire structure
Now that we have seen how this solution works, letās apply it to larger structures with different diameters of rebar.Ā
We have prepared a table with the demand from the design documentation:
To see the full code and results, you can refer to the Jupyter notebook available at the following link:Ā https://nbviewer.org/github/inigmat/exupery/blob/main/CSP_binpacking.ipynb
After optimization, we obtained the following results for the required number of reinforcement bars and the total scrap percentage for each diameter:
Required number of reinforcement bars with diameter 10 mm: 168 pcs
Total scrap: 2.32%
Required number of reinforcement bars with diameter 12 mm: 138 pcs.
Total scrap: 1.47%
Required number of reinforcement bars with diameter 16 mm: 143 pcs.
Total scrap: 3.76%
Required number of reinforcement bars with diameter 20 mm: 759 pcs.
Total scrap: 4.82%.
We also generated a chart showing the cutting plan for the bars.
For a more detailed view of the data: link
Part 3. Looking under the hood
While optimizing the cutting of items from larger pieces to satisfy the demand and minimize waste, we are actually solving theĀ Cutting Stock ProblemĀ (CSP). This problem involves finding the most efficient way to cut larger pieces into smaller items, considering constraints such as item sizes and quantities. CSP is a variant of theĀ packing problem, specificallyĀ bin packing problem, which aims to find the fewest bins that can hold all the items. This is why we use the ābinpackingā package, as it is designed to address this problem.
The main feature of theĀ ābinpackingāĀ Ā package is theĀ first-fit decreasing algorithm, which is much faster than solutions based onĀ mixed-integer programmingĀ when dealing with a large number of elements.
More read here:
Conclusion
By implementing the code presented in this article, we can find the optimal quantity of materials to order for a given project. The approach provides flexibility and additional features compared to online calculators. With the help of the ābinpackingā package, we can efficiently solve the Cutting Stock Problem.
Whether you are working on a small-scale project or a larger construction endeavor, this method can help save time, reduce waste, and ensure efficient material usage.